03.What Are Data Types in Python? Simple Explanations with Code Examples
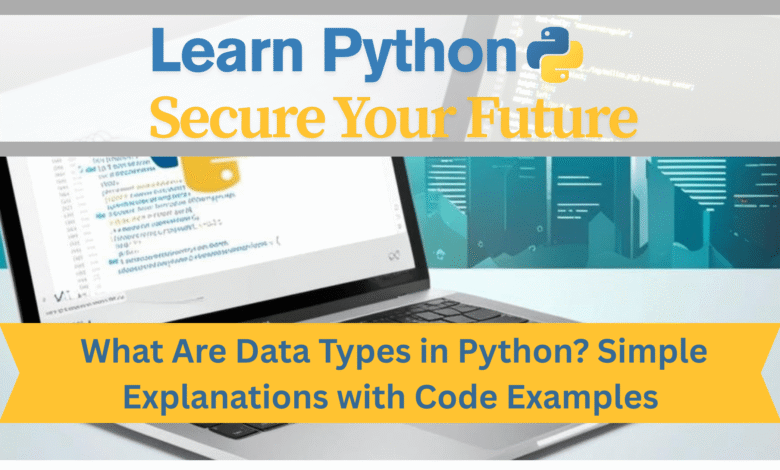
Definition:
A data type is made of two words: “data” and “type”. It tells us about the kind of data we are dealing with. Suppose if we do not tell the type of data we are using, then everything would be mixed up and will create confusion. We would not know which variable holds what kind of data. This can lead to errors and unexpected behavior in our program.
Python is a dynamically typed language. This means we do not have to declare the type of variable before using it. Python will automatically understand what type of data we are assigning to a variable. But still, we study data types so that we can use them correctly in our programs. It’s important to understand how data types work to write clean, reliable, and efficient code.
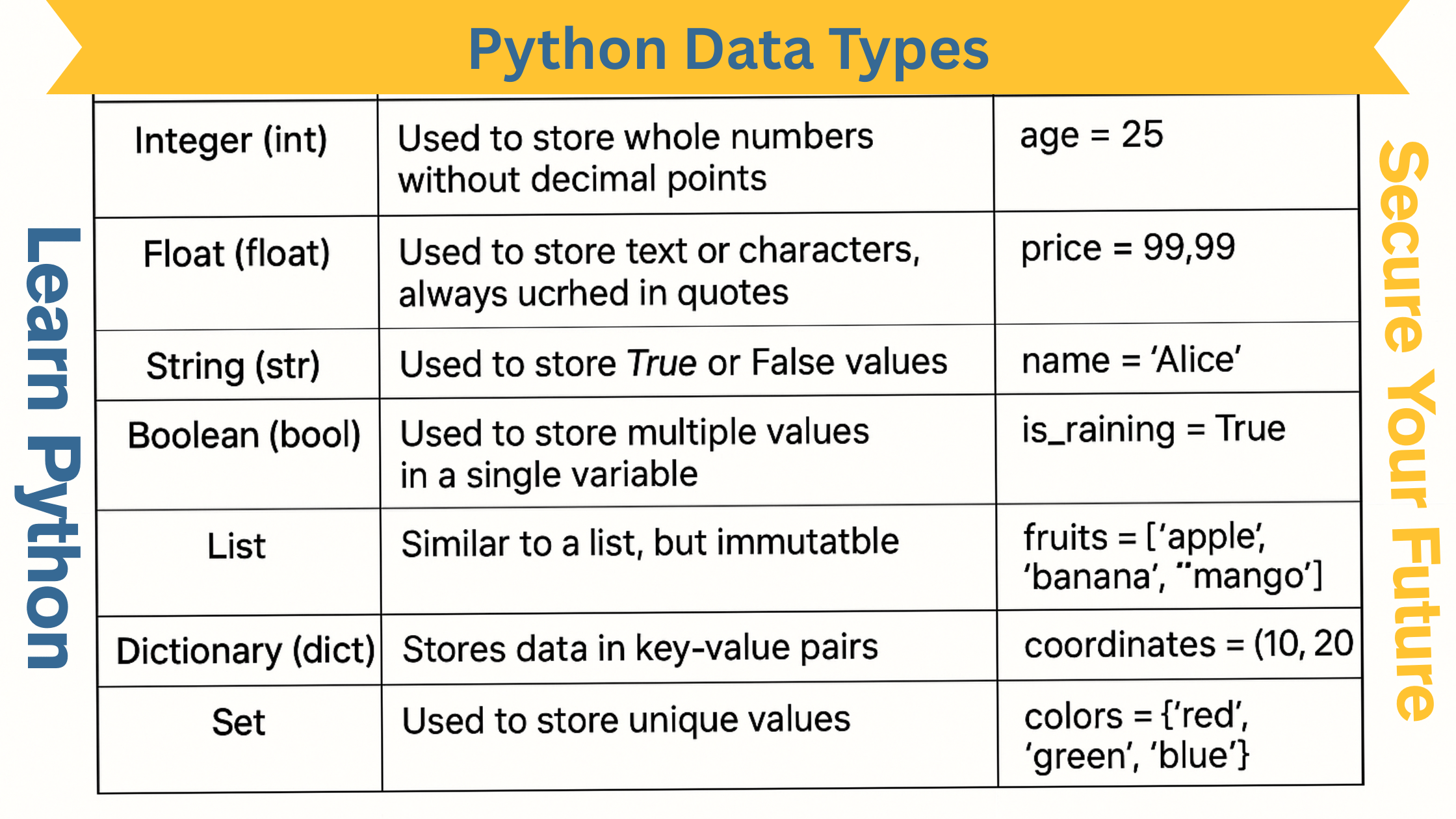
Now let’s understand the different types of data used in Python, one by one:
1. Integer (int)
The integer data type is used to store whole numbers (numbers without any decimal points). These numbers can be positive or negative. We use integers when we need to count something or store numeric values that do not require fractions. For example, if we are storing someone’s age, we do not need decimals, so we use an integer.
Example:
age = 25
Here, 25
is a whole number, and Python automatically understands that it is an integer.
2. Float (float)
Float stands for “floating-point number,” and it is used to store numbers that have decimal points. These numbers can also be positive or negative. When we need more precision, like dealing with prices, temperatures, or measurements, we use float.
Example:
temperature = 36.5
In this case, 36.5
is a float because it includes a decimal part. Python knows that it’s not a whole number and treats it as a float.
3. String (str)
A string is used to store a sequence of characters. It can include letters, numbers, spaces, and symbols. Strings are always written inside quotes (either single or double quotes). We use strings when we want to store names, messages, sentences, or any kind of text.
Example:
name = "Aamir"
Here, "Aamir"
is a string because it is text written inside quotes.
4. Boolean (bool)
Boolean data type is used to represent truth values (either True or False). It helps in making decisions in our code using conditions. Booleans are usually the result of comparison operations or used in if-else statements.
Example:
is_sunny = True
In this example, is_sunny
is storing a True value. This helps the program decide what to do next based on the weather.
5. List
A list is a collection of items stored in a single variable. Lists are written in square brackets []
, and the items inside can be of different data types. You can also change, add, or remove items in a list. We use lists when we want to store multiple values like a group of names, numbers, or objects.
Example:
fruits = ["apple", "banana", "mango"]
Here, fruits
is a list containing three string items. We can access them using their position in the list (called index).
6. Tuple
A tuple is similar to a list, but once you create it, you cannot change its items. Tuples are written in round brackets ()
. We use tuples when we want to store a collection of items that should not be changed throughout the program.
Example:
coordinates = (10, 20)
This is a tuple with two numbers. It is useful when we want to lock the values and keep them safe from accidental changes.
7. Dictionary (dict)
A dictionary stores data in key-value pairs. Each key is connected to a value, and we use the key to access the value. Dictionaries are written in curly braces {}
. We use dictionaries when we want to store related information like the details of a student or product.
Example:
student = {"name": "Ali", "age": 20}
Here, "name"
and "age"
are keys, and their values are "Ali"
and 20
. This is a good way to keep structured data.
8. Set
A set is a collection of unique items. This means it does not allow duplicate values. Sets are also written in curly braces {}
, but they only store values, not key-value pairs like dictionaries. We use sets when we want to make sure all items are different.
Example:
colors = {"red", "green", "blue"}
In this set, even if we try to add "red"
again, Python will ignore the duplicate because sets only allow unique values.
These are the basic data types in Python. Knowing them well helps us write better and more understandable code. Even though Python detects the type automatically, understanding how each one works is important for solving real-world problems with programming.
Also Read: Understanding Variables in Python: The Ultimate Beginner’s Guide (With Examples & Tips)
Also Read: The Story Behind Python, Why the World Fell in Love with Python (2025)?